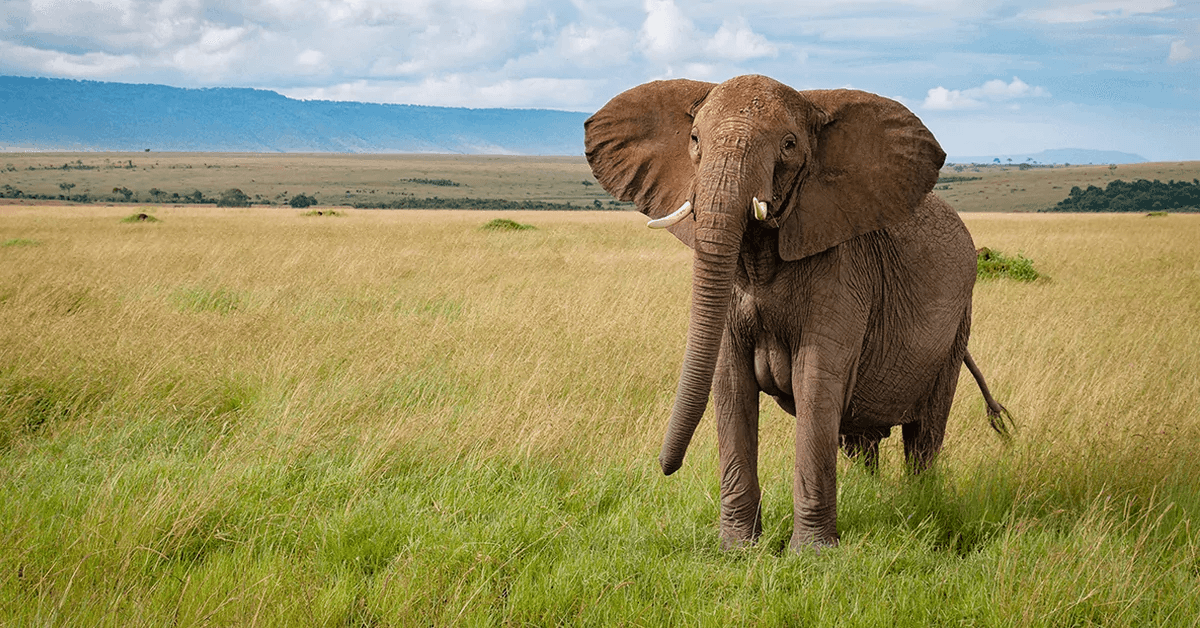
Table of Contents
This article’s sole purpose is to quickly show you how to send SMS using PHP.
Send SMS Using PHP: A Step-By-Step Guide
If you’re a developer looking to incorporate SMS functionality into your PHP applications, you’re in the right place. With the SMS API in PHP, you can seamlessly integrate SMS capabilities into your web or mobile apps. Whether you need to send one-time verification codes, notifications, or promotional messages, using a reliable short message service gateway simplifies the process. By leveraging PHP, a popular server-side scripting language, you can create dynamic and interactive SMS-driven experiences for your users.
See how to send bulk SMS messages, customize content and schedule messaging in one of the most popular programming languages for applications, websites. Nothing less, nothing more than a well-documented SMS API for business communication.
SMS API: PHP Library
We have been developing our PHP library since 2013. A stable, reliable and open API allows us to provide developers with a wide range of tools. Most of them are ready to use straight from the box, our Documentation. No need to write every tiny detail by yourself, right? This is probably the easiest (and the most secure) way to use the bulk SMS messaging platform. Let’s send some text messages using PHP!
Yet before we go any further, keep in mind that you can always fall back to the API. It has everything you might need to at least start with a professional A2P SMS communication channel. Furthermore, there are also practical examples in different languages, like C#, Bash, and Python. PHP is one such implementation.
If you wish to look closely at the Library, its uses and other details, visit the SMSAPI PHP repository on GitHub. As usual, the source code is probably the best source of knowledge, offering insight into all functionalities. Were you looking for a more pragmatic approach? Stick around. I’ll go in-depth on everything you need to start sending SMS with PHP from web.
Sending SMS messages using PHP is a powerful way to engage with your audience. In this step-by-step guide, I’ll walk you through the process of sending SMS messages from your PHP application. Send SMS using PHP and automate notifications, alerts, or marketing campaigns.
I’ll cover everything from setting up your SMS gateway to crafting and delivering messages programmatically. With just a few lines of code, you’ll be able to reach your users via SMS, enhancing your application’s functionality. Learn how to optimize your PHP code for mass messaging while ensuring delivery reliability. From database integration to message scheduling, we’ll cover the essential techniques for successful bulk SMS messages.
Integrated SMS API in PHP: first steps
First things first, you’ll need an access to SMS gateway: SMSAPI account. Furthermore, you better configure it immediately—it’ll save a hassle later.
How to configure the SMSAPI account
- Create a SMSAPI account – get it free, with test messages included.
- Fill in the company details in the Payments section – don’t worry, tests are free. We use your data for account verification purposes only.
- Once verified, you’ll be granted a free SMS quota and the ability to set your SMS sender names.
- Generate API token. You will use this unique, random 40-character long string to authenticate the connection. The HTTP client sends the authorization request header when accessing a restricted API.
Watch video tutorials and do it yourself
Straight to the point: how to send SMS with PHP?
Relax, I won’t describe every class and method. Though I’ll focus on what I think is entry-level knowledge, you’ll be able to do the rest yourself—starting with the SMS notification system. Let’s go!
PHP SMS gateway
SMS messaging is a core of the SMSAPI gateway. You can implement the message composing and database management however you like, but you will need an SMS API to send the message. How does it work code-wise?
As SMSAPI is a RESTfull API, you must set up an HTTP client to utilize its methods. The PHP code allows you to use the provided libcurl library adapter, so you don’t have to implement your HTTP client—but if you wish, do so. It’s easier to use the stock solution. Here’s how you can start:
<?php
//client.php
declare(strict_types=1);
use Smsapi\Client\Curl\SmsapiHttpClient;
require_once 'vendor/autoload.php';
$client = new SmsapiHttpClient();
With a client prepped and ready, connect to https://api.smsapi.com as shown in the second file listing:
<?php
//service.php
declare(strict_types=1);
use Smsapi\Client\SmsapiClient;
require_once 'vendor/autoload.php';
require_once 'client.php';
$apiToken = '%SMSAPI_ACCESS_TOKEN%';
$service = $client->smsapiComService($apiToken);
Two simple lines (not counting the namespace calls) are enough to create SmsapiHttpClient
and smsapiComService
objects. This concludes the basic configuration. So far, so good.
SMS messaging via PHP
The SmsFeature
interface implements SMS messaging. Here are its key methods:
sendSms(SendSmsBag $sendSmsBag)
– send an SMS message to a single contact;sendSmss(SendSmssBag $sendSmssBag)
– send SMS messages to multiple contacts.
The “Bag” type objects determine elements of a text message. Their properties are recipient or recipients, content, and other optional parameters. Find the practical example below:
<?php
//sendSms.php
declare(strict_types=1);
use Smsapi\Client\Service\SmsapiComService;
use Smsapi\Client\Feature\Sms\Bag\SendSmsBag;
require_once 'service.php';
$smsBag = SendSmsBag::withMessage('44111222333', 'Hello world!');
$service->smsFeature()->sendSms($smsBag);
The withMessage
factory method takes two string arguments: recipient phone number (plus country code) and message body. In a nutshell, sending messages using the PHP is done in four simple steps:
- creation of the HTTP client;
- connection with the short message service,
- definition of a message body and recipient,
- message delivery.
Additional features of PHP SMS notifications
The sendSms method returns the SMS object containing a bunch of helpful information:
$id
– id number in SMSAPI;$points
– message price presented as the points deducted from your SMSAPI account;$dateSent
– time of messaging, UNIX timestamp format;$status
– delivery status (complete list and explanation).
There’s a huge chance that your SMS communication will depend on delivering the same messages to many different recipients. Easy-peasy, use the sendSmss
function and SendSmssBag
class.
Alternatively, create groups in the Contacts tab and send a bulk SMS campaign like this:
$receivers = ['44111222333','44444555666'];
$smssBag = SendSmssBag::withMessage($receivers, 'Hello, receivers!');
$service->smsFeature()->sendSmss($smssBag);
$smsToGroupBag = SendSmsToGroupBag::withMessage('group_name', 'Good morning, contact group!');
$service->smsFeature()->sendSmsToGroup($smsToGroupBag);
Timely messaging – SMS scheduling
The message scheduling feature will be essential for larger repeatable campaigns. After all, repetition draws attention. But to do it right, you must determine the proper time and frequency first. Thus I recommend A/B tests to find your sweet spot!
The PHP to send SMS notifications comes with multiple schedule functions. As expected, the API will unfailingly deliver messages at a designated time for you, so you don’t have to implement such a program yourself.
The code below presents it in three ways as mentioned earlier: single message, bulk campaign and messages sent to a contact group:
$sendingDate = new DateTime('2027-06-30');
$scheduleSmsBag = ScheduleSmsBag::withMessage(
$sendingDate,
'44111222333',
'An SMS is never late, nor is it early. It arrives precisely when it means to.');
$smsFeature->scheduleSms($scheduleSmsBag);
$scheduleSmssBag = ScheduleSmssBag::withMessage(
$sendingDate,
['44111222333', '44444555666'],
'An SMS is never late, nor is it early. It arrives precisely when it means to.');
$smsFeature->scheduleSmss($scheduleSmssBag);
$scheduleSmsToGroupBag = ScheduleSmsToGroupBag::withMessage(
$sendingDate,
'group_name',
'An SMS is never late, nor is it early. It arrives precisely when it means to.');
$smsFeature->scheduleSmsToGroup($scheduleSmsToGroupBag);
SMS personalization in PHP library: parameters and message templates
If you are thinking about a bulk SMS campaign, you should also be thinking about content personalization. Otherwise, you would be sending the same message to every recipient. And you can do better than that! Use the following two static methods for every “Bag” type object to be personal and, not to mention, way more effective.
The first method, namely setParams(array $params)
, allows you to use any of the following parameters: $param1
, $param2
, $param3
, $param4
. These are additional properties for the Bag class. Function’s argument is a key array ([%1%]
, [%2%]
, [%3%
], [%4%]
) that will be replaced with the values ‘1’, ‘2’, ‘3’, ‘4’ in the message body. Here’s a code snippet:
$receivers = ['44111222333','44444555666'];
$params = [
1 => ['Suzy', 'Barbra'],
2 => ['London', 'Innsmouth'],
];
$smssBag = SendSmssBag::withMessage(
$receivers,
'Hello [%1%] from [%2%]!'
);
$smssBag->setParams($params);
$service->smsFeature()->sendSmss($smssBag);
The second method, withTemplateName(string $receiver, string $template)
, allows you to use templates created in the Customer Portal. It is convenient because you can change message content on the fly without changes to your PHP code. The SMS template might look like this:
- PackageSentTemplate: Hi [%1%]! Your order is on its way!
- PackageArrivesTodayTemplate: Hi [%1%]! Your order will arrive today!
And messaging like this:
$params = [1 => 'Adam'];
$smsBag = SendSmsBag::withTemplateName(
'44111222333',
'PackageSentTemplate'
);
$smsBag->setParams($params);
$service->smsFeature()->sendSms($smsBag);
$smsBag = SendSmsBag::withTemplateName(
'44111222333',
'PackageArrivesTodayTemplate'
);
$smsBag->setParams($params);
$service->smsFeature()->sendSms($smsBag);
Further content personalization using a contact database
One way to improve your text message is to deliver relevant information to each recipient. The PHP library can access and use the database to automatically personalize the content of your messages. Hence there’s no need to put the recipient’s data in the PHP script. SMSAPI has a function to browse and filter a database with an e-mail address, etc. This allows you to send messages containing user-specific data, like the one below about a reservation:
- TableReservationTemplate: Hello [%name%]! Your table at Embers will be ready at [%1%]. Our chief recommends a liver with some fava beans and a nice Chianti!
Here’s how to personalize SMS in PHP:
use Smsapi\Client\Service\SmsapiComService;
use Smsapi\Client\Feature\Sms\Bag\SendSmsBag;
use Smsapi\Client\Feature\Contacts\Bag\FindContactsBag;
use Smsapi\Client\Feature\Contacts\ContactsFeature;
require_once 'service.php';
$receiverEmail = '1234@example.com';
$reservationTime = '19:00';
$contactFindBag = new FindContactsBag();
$contactFindBag->email = $receiverEmail;
$foundContacts =
$service->contactsFeature()->findContacts($contactFindBag);
$smsBag = SendSmsBag::withTemplateName(
$foundContacts[0]->phoneNumber,
'TableReservationTemplate'
);
$smsBag->setParams([1=>$reservationTime]);
$service->smsFeature()->sendSms($smsBag);
An e-mail or any other information can help you retrieve a phone number, then personalize a message and send an SMS. For example, the [%name%]
field will be replaced with the actual name of your customer from the contact database. Refer to the Documentation for further information on personalized SMS messages.
PHP SMS API: Enhance User Engagement
Incorporating a PHP SMS API into your applications is a game-changer for user engagement. With the short message service center, you can create a dynamic user experience. Harness the power of the SMS API in PHP to trigger SMS alerts based on user actions, such as account activities or in-app events. By integrating PHP and an SMS API, you can keep your users informed, drive conversions, and enhance overall satisfaction with your application.
SMS sender PHP’s ready. What’s next?
Go to GitHub if you are looking for the source code for project. You will find language-specific SMSAPI libraries for Java, C#, Python, bash, and JavaScript clients there.
This introduction focuses on the small portion of possible applications of the PHP script. There’s a lot more, and most can be found in the Feature folder, e.g.:
- multi-factor authentication (MFA) to make the logins more secure;
- contact database management – add, edit and delete contacts and contact groups;
- the cut.li link shortener tool;
- account balance checkup – get remaining points for outgoing messages in PHP.
Lastly, check the test folder when needing more interfaces and methods examples. Good luck!