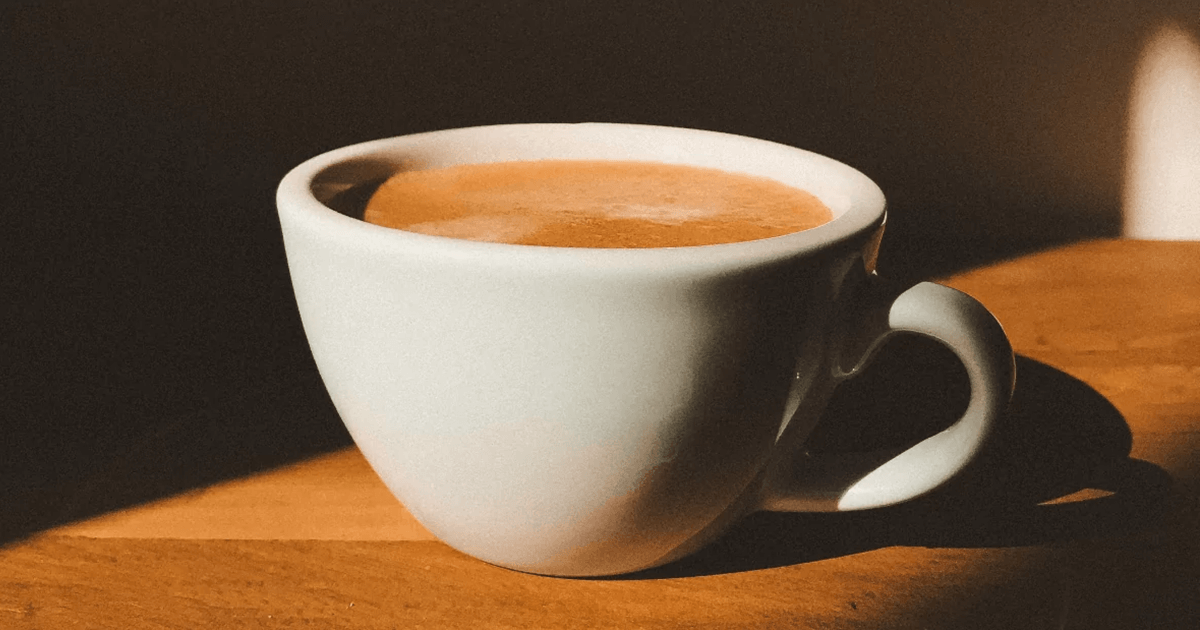
Table of Contents
Here’s your go-to guide for Java SMS messaging. Introducing SMS API Java Library, along with code snippets, step-by-step instructions, and an explanation of the most common errors.
Sending SMS Messages in Java: Practical Tips and Techniques
Sending text messages using Java is an essential feature for many applications. And this article provides practical insights and techniques on how to so. Discover the best practices for integrating SMS functionality into your Java applications, including handling message templates, managing recipient lists, and ensuring message delivery. Whether you’re building a messaging app or enhancing user communication, these tips will help you streamline the process.
For many years, Java has reigned as one of the most common programming languages in the world. SMSAPI meets this trend by providing a ready-made Java library for handling SMS messaging. So if your programs are written in this language, read on!
As with all other SMS API libraries, this one is publicly available on GitHub. View the source code there. This, of course, is the surest source of information on its functions and capabilities. In this guide, however, I’ll discuss the Java library in a much friendlier manner so that creating a Java application is simpler than making yourself a coffee. Let’s go!
Installing the library for handling SMS capabilities in Java
First, attach the Java library files to your project. You can automate this using the Maven tool. All you need to add in the POM configuration file is one element to the repository and dependencies lists.
<repositories>
<repository>
<releases>
<enabled>true</enabled>
<updatePolicy>always</updatePolicy>
<checksumPolicy>fail</checksumPolicy>
</releases>
<id>smsapi</id>
<name>smsapi</name>
<url>https://labs.smsapi.com/maven/</url>
<layout>default</layout>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>com.smsapi</groupId>
<artifactId>smsapi-lib</artifactId>
<version>2.5</version>
</dependency>
</dependencies>
On the SMSAPI server, you’ll find library versions from 1.1 all the way up to 2.5. These contain JAR archives with the source code, compiled byte code, detailed documentation generated by Javadoc, and the POM files.
If you chose the Gradle tool to manage your app’s development, then using the SMSAPI library in Java will be just as easy. Apply the Java plugins and their libraries to the build.gradle file:
plugins {
id 'java'
id 'java-library'
}
Include the SMSAPI library in the repositories:
repositories {
mavenCentral()
maven {
url 'https://labs.smsapi.com/maven/'
}
}
The last thing to do is to import Java library in the right version, depending on your project:
dependencies {
api 'com.smsapi:smsapi-lib:2.5'
}
Send an SMS using Java application: configuration
Before you begin the coding, there’s one more essential thing you need to do: set up access to SMS API. Create a trial account. It’s free and comes with a free SMS quota for tests. Or log in to yours.
Test SMSAPI
Are you looking for a professional SMS gateway for your Java applications? You are in the right place! Test our messaging service, open and well-documented SMS API and start sending SMS messages to many countries.
This will allow us to start working together. The Customer Portal is a graphical interface with which you can manually handle your entire SMS campaigns. Our Portal has tons of productive functions, i.e., sending messages, receiving incoming communication, HLR lookup and more. You will find there the account settings, MMS messages sending, recipient database and sendername management, as well as other options. Go through the SMSAPI Do It Yourself guide if you’d like to know the Portal inside out.
The API is a RESTfull gateway that allows you to post messages, find out their state and much more. To communicate with server you will send HTTP requests using HTTP methods: POST, GET, PUT and DELETE. Send HTTP GET reqyest to contacts endpoint to find out what contacts you already have collected, for example. In the library code, they are handled using the Proxy
package via the Client
interface. It is the library’s backstage of sorts, you don’t need to learn it in too much detail.
To establish a secure connection with SMS and MMS messages gateway, you need a 40-character API OAuth2 token. Setting up a client looks something like this:
import com.smsapi.OAuthClient;
import com.smsapi.proxy.Proxy;
import com.smsapi.proxy.ProxyNative;
class Main{
public static void main(String[] args){
String token = "%SMSAPI_ACCESS_TOKEN%";
var client = new OAuthClient(token);
var proxy = new ProxyNative("https://api.smsapi.pl/");
}
}
As you can see, a server connection also requires a proxy object. It routes all requests to https://api.smsapi.com/ domain. However, you can skip an overt generation of the proxy. It will then be created by default, connecting to the api.smsapi.pl service.
Time to send SMS, Java-style!
Time for the real stuff! Our library relies on factories and actions to send text messages. The former corresponds to individual functions, allowing you to set their parameters.
Every action should begin with creating the factory and end with calling the execute()
function from the base Java class. Of course, once a factory is created, you can later use it as often as you want. To expand the previous listing with simple SMS sending (a proxy is created by default):
import com.smsapi.OAuthClient;
import com.smsapi.api.SmsFactory;
import com.smsapi.api.action.sms.SMSSend;
class Main{
public static void main(String[] args){
var client = new OAuthClient("%SMSAPI_ACCESS_TOKEN%");
var smsFactory = new SmsFactory(client);
var actionSmsSend = smsFactory.actionSend("+32111222333", "Hello world!");
actionSmsSend.execute();
}
}
It can be useful to chain-link methods from action classes. The fragment below works the same way:
var smsFactory = new SmsFactory(client);
smsFactory.actionSend()
.setTo("+32111222333")
.setText("Hello world!")
.execute();
The Java library: bulk sending SMS messages
Performing bulk SMS sending wouldn’t be possible without the ability to send messages to multiple recipients at the same time. The SmsFactory
factory makes it very simple thanks to overloading the actionSend
and setTo
methods:
SendStatusResponse response = smsFactory.actionSend(
new String[]{"+32111222333", "+32444555666"},
"Hello receivers!")
.execute();
SendStatusResponse response = smsFactory.actionSend()
.setTo(new String[]{"+32111222333", "+32444555666"})
.setText("Hello again receivers!")
.execute();
That’s not all, though. In the Customer Portal, you’ll notice that recipients can be joined into groups. Sending identical SMS notifications to all group members is very simple:
SendStatusResponse response = smsFactory.actionSend()
.setGroup("Group_name")
.setText("Hello group!")
.execute();
The above listings retain the object returned by the execute()
method. In this case, it is the SendStatusResponse
class. It has the following methods:
getParts()
– returns the number of parts that an SMS has been divided into. Content longer than 160 characters might require splitting into two or three SMS text messages.getCount()
– returns the number of messages queued to be sent.getList()
– returns a list (ArrayList) of objects of class MessageResponse. Its methods, in turn, return information on individual SMS sent. These are:getId()
– a unique text message identifier in the system.getPoints()
– message cost expressed in points deducted from your account.getNumber()
– recipient’s mobile phone number.getStatus()
– one of the delivery statuses.
Scheduling SMS in Java project
There are a few more practical functions of the library that are worth a mention. The first is scheduling SMS using Java methods. The right date and time when a notification is delivered to your clients are crucial to elicit the right reaction. How? Check out the article below.
You can easily set the time in your code using the setDateSent methods from the SMSSend
action. They accept either Calendar
object or a number of seconds from the start of the epoch. This example sets the messages to be sent on 21 January 2023 at 12:00 p.m.
import java.util.GregorianCalendar;
var sendDate = new GregorianCalendar(2023, 0, 1, 12, 0);
SendStatusResponse response = smsFactory.actionSend()
.setTo("+328111222333")
.setText("Hello in the future!")
.setDateSent(sendDate)
.execute();
In the example below, on the other hand, provided phone number will receive SMS message in an hour:
import java.util.Date;
var sendDate = new Date().getTime()/1000 + 3600;
SendStatusResponse response = smsFactory.actionSend()
.setTo("+32111222333")
.setText("Hello in the future!")
.setDateSent(sendDate)
.execute();
Using this method, you can set the date up to 3 months ahead. If you change your plans during this time, remove the SMS from the schedule using the SMSDelete
action. To do it, you need the previously-mentioned unique ID of a scheduled SMS. This looks, for example, as follows:
String smsIdToDelete = response.getList().get(0).getId()
smsFactory.actionDelete(smsIdToDelete).execute();
From the object of class SendStatusResponse
you take the list of SMS sent, and from the list, you get the zero elements, and from that, you get the ID. With a method from the actionDelete the factory, you create an action, provide a parameter for it right away, and execute it.
Java: send SMS messages that make an impact
Unique, engaging content is paramount. The library provides two simple tools to make your SMS marketing and notifications stand out.
One is the SMS sendername (or SMS branding) – a basic and free-of-charge function of the SMS gateway. It is a short text displayed on the mobile phone screen instead of a phone number. The name should somehow identify your company, brand or product so that a person can tell at a glance who’s texting them. Request yours in the Customer Portal, but remember we do check each one and spam and scam messaging are not allowed.
This method of drawing the recipient’s attention is simple but useful in SMS marketing. SMS sender names can be managed manually using the relevant section of the Customer Portal. In the code, you can use an existing name using a method from the SMSSend
action to send SMS with unique sender id:
smsFactory.actionSend()
.setTo("+32111222333")
.setText("Hello world!")
.setSender("sender_name")
.execute();
The other method is message parametrisation. Special character strings in the SMS text allow you to customise bulk-sent messages. The SMS system will fill messages with data corresponding to the phone numbers.
This way, they won’t be identical but will address the recipients more individually. You use four custom names and the name values from the contact database. They are described in detail in the Documentation. Let’s take a look at an example:
smsFactory.actionSend()
.setTo(new String[]{"+32111222333", "+32444555666"})
.setText("Hello [%first_name%], your number is [%1%]!")
.setParam(0, new String[]{"11", "22"})
.execute();
The texts of all (two) SMS will be modified twice. The [%first_name%] names will be replaced with the corresponding values from the contact database.
The setParam
method provides the replacement for name [%1%]. Its first argument is the parameter index, the second is an array of strings inserted into consecutive messages instead of the special strings. There are four such custom parameters available: [%1%], [%2%], [%3%], and [%4%], corresponding to indexes 0, 1, 2, 3.
Handling SMS sender names in the SMSAPI Java library
As I’ve mentioned above, it’s worth using the SMS sendername feature. On the program side, they are handled by SenderFactory
and action classes: SenderList
, SenderAdd
, SenderDefault
, SenderDelete
.
The example below shows how to call a list of existing SMS sendernames, add a new name, set it as default and delete it. I assume that a client object (which the factory needs) has already been set up.
var senderNameFactory = new SenderFactory(client);
SendersResponse response = senderNameFactory.actionList().execute();
for (SenderResponse elem: response.getList()){
System.out.println(
"Sender name: "+elem.getName()
+"Sender status: "+elem.getStatus()
+"is default?: "+elem.isDefault()
);
}
senderNameFactory.actionAdd("NewSenderName").execute();
senderNameFactory.actionSetDefault("NewSenderName").execute();
senderNameFactory.actionDelete("NewSenderName").execute();
The message SMS sender name ID must meet certain requirements. The most important thing is to remember that for security reasons, each new name must be approved by our employee during SMSAPI business hours. Verify your account first, it’ll speed up the process!
Free tests, no strings attached
Create a free trial account, no credit card required. Send test message in minutes and see other advanced features of business SMS gateway.
Error handling in the SMSAPI Java library
During automatic SMS handling, errors may occur in many places. This may occur, for example, when connecting to the server, constructing queries, applying various parameters, or processing server responses. The server sends an error code and message instead of the expected response when something goes wrong.
You can find a detailed list of codes in the Documentation. It’s worth protecting yourself against errors, which is why the library defines multiple exception classes.
ClientException
– these exceptions concern HTTP methods. The error codes used are, for example, 101 (incorrect OAuth2 token) and 103 (insufficient points to send the SMS).HostException
– these mean errors on the SMSAPI server’s side. The codes include 8 (call error) and 201 or 999 (internal system error).ActionException
– these exceptions result from the incorrect use of one of the actions. Error code 11 is too long or has no message text. 13 is an incorrect recipient number or means a group and individual number are used simultaneously. 14 means an incorrect sender ID; 40 means the specified group does not exist.ProxyException
– these occur when a server connection error happens. They have no code from SMSAPI. They are thrown when an exception of the java.io.IOException class occurs.
Specific error codes are returned by the getCode()
method. In one case, if a message parameter with an index other than 0, 1, 2 or 3 was attempted to be called, an index out of array exception (ArrayIndexOutOfBoundsException
) is also thrown.
The SMSAPI Java library – more options
There are also other factories not described above which offer further possibilities for your Java app. One of them is ContactsFactory
– as the name suggests, it is used to handle contact databases. It allows you to list, delete or edit individual recipients and their groups. Creating a new entry may look something like this – assuming there already is an HTTP client object:
ContactsFactory contactsFactory = new ContactsFactory(client);
contactsFactory.actionContactAdd()
.setFirstName("Test First Name")
.setLastName("Test Last Name")
.setPhoneNumber(numberTest)
.setEmail("test@example.com")
.execute();
Send MMS messages with Java
Another factory, called MMSFactory
, is used to handle multimedia messages. The key is its actionSend method, which works the same way as for SMS sending, described earlier.
Executing it (using the execute()
method) returns a SendStatusResponse
object, and with the “set” methods, you can set the message parameters, such as recipients and send date. Instead of a regular text, you must deliver a message in the SMIL format and its subject. The example below assumes that such correct content already exists:
mmsFactory = new MMSFactory(client);
mmsFactory.actionSend()
.setTo("+32111222333")
.setSubject("test_subject")
.setSmil(smil_content)
.execute();
As we’re nearing the end, I’ll point you to a few places where you can seek answers to any technical questions you may have. The first is the API documentation, which has already been mentioned several times. There are no references to Java code there, but it contains many detailed explanations. They’ll help you understand what individual parameters, error codes and the ways that different features work are intended to do.
If you find Javadoc documentation convenient to use, download the latest version (2.5) from the SMSAPI server. Clearly structured and with lots of comments, it will be much easier to find your way around all the available classes, methods and packages.
Unit tests, written using JUnit, are a good source of information too. You’ll find them on GitHub. When the library was created, they were used for quality assurance, and it’ll provide you with examples of using individual interfaces and methods.
If this doesn’t clarify all the points you’re uncertain about, dive into the most complete knowledge source of all, the library code. And don’t forget – our technical support is here to help you. Good luck!
Oh, one more thing: I’ve covered how to receive SMS messages in a separate article.