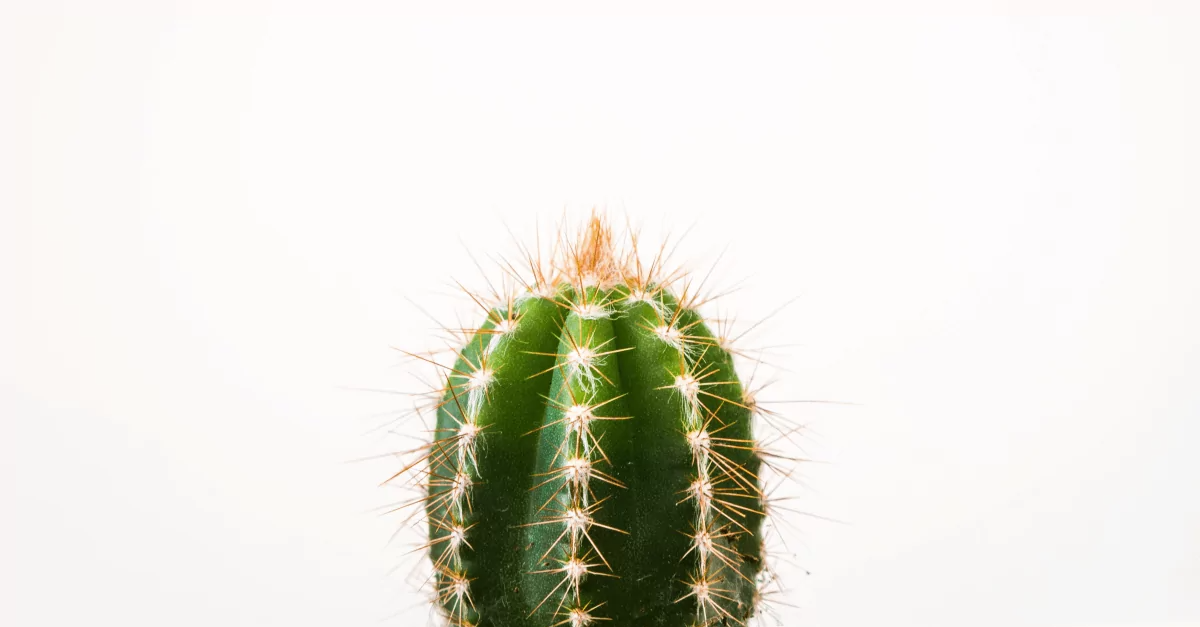
Table of Contents
How to send SMS messages from C#? Beginning with the basics, get to know our SMS API library, including sample code, practical advice, solutions to common problems, and more.
Time for an introduction to another library for bulk SMS gateway for developers! In addition to PHP, Java, JavaScript and Python discussed previously, SMSAPI also offers you implementation in C#. If this is a fit for your programming tastes – read on!
The entire source code is made public on SMSAPI GitHub. There you will find two folders:
- smsapi – containing the production code you can use in your application. You’ll learn about its selected methods below.
- smsapiTests – having unit tests which utilise the MSTest framework. Here you can find examples of the individual methods.
Test SMSAPI
Still looking for the best SMS API? Create a test account and give it a shot!
The entire Library implements the API described in the SMSAPI Documentation. If you look there, you will undoubtedly find many analogies to the objects and methods in C#. For your convenience, we’ve illustrated it with numerous examples of how to send text messages.
You can, of course, write your app from scratch in your chosen language, referring to the API directly. But it’s much simpler to copy and pa… pardon, use a ready-made solution. Let’s send SMS already!
Create SMSAPI account
As with any other use of API services to handle SMS messages, register and then configure your account. You’ll need to provide the following data:
- basic info – e-mail (username), password (keep it private), phone number, first and last name;
- company details – for invoicing;
- SMS sender name – not mandatory, although useful;
- additionally – an e-mail address for sending invoices and a contact phone number to your accounting department.
After verification, we’ll activate your account. Start with charging your account with points for messaging! For more, see the SMSAPI Do It Yourself series – a comprehensive guide to a professional SMS gateway. Here we’ll focus on programming SMS using C#.
First steps in handling SMS campaign: configuration
Behind the scenes of our Library operates the RestSharp
library that handles HTTP requests. It uses HTTP (GET, POST, PUT, DELETE) methods to handle all server communication and the text message campaign. Luckily for you, you don’t have to get into the details to use it.
Where and how to start, then?
As with most other projects: from the initialisation. The connection should be authorised using an API token. It is a string of 40 characters, which you can generate in the API Tokens (OAuth) tab and, subsequently, use in Visual Studio:
SMSApi.Api.IClient client = new SMSApi.Api.ClientOAuth("token");
The client
objects store data later used in authorisation.
SMS message sending using C# methods
Once a client is set up, the Library works based on factories and actions, as shown in the example below. Here, a test message sent to a single recipient:
var smsFactory = new SMSApi.Api.SMSFactory(client);
SMSFactory.ActionSend("48111222333", "Hello world!").Execute();
Three lines are enough to include the three basic stages of simple SMS handling: creating a client, creating a factory, and applying the right action. The server connection is handled within an object of the Factory
class, which serves as a basis for numerous factories, including SMSFactory
. These are responsible for the individual functions of the Library.
On the other hand, methods of the Action type allow you to set up individual parameters of actions you want to perform. Method calls can be chained, starting from the Library and ending with execution (the Execute()
method from the base class of all actions). For example, the following code functions in the same way as in the previous project:
var smsFactory = new SMSApi.Api.SMSFactory(client);
var response = SMSFactory.ActionSend()
.SetTo("48111222333")
.SetText("Hello world!")
.Execute();
Responses to different queries to the API handle classes from the Response
namespace. When ordering SMS sending in C#, use Response.Status
(object type response
from the example above). It has several useful properties. The count
is the number of messages sent. The list
is a list of their corresponding variables, including:
ID
– a unique identifier in the SMSAPI system.Points
– cost expressed in the number of points deducted from your account.Number
– user phone number.Status
– delivery status (see the status list in the Documentation).
Other properties: Message
, Length
and Parts
are, respectively, the content, number of characters used and parts of the text.
Send bulk SMS sending in C# code
The most basic function is sending the same text message to multiple recipients at once. Typing separate messages to each of your clients would simply be too work-intensive. By overloading the ActionSend()
and SetTo()
methods, this is handled by a minor code change.
This is what sending identical messages to three recipients in one of two ways looks like SMSFactory
and using method chaining from the SMSSend
action:
SMSFactory.ActionSend(
new[]{"48111222333", "48444555666", "48777888999"},
"Hello receivers!")
.Execute();
SMSFactory.ActionSend()
.SetTo(new[]{"48111222333", "48444555666", "48777888999"})
.SetText("Hello again receivers!")
.Execute();
In the Customer Portal, you’ll notice the ability to create recipient groups. The C# library allows you to use them to streamline bulk SMS campaign management. Another fragment showcases this:
SMSFactory.ActionSend()
.SetGroup("Group_name")
.SetText("Hello group!")
.Execute();
Automated SMS in C# – additional options
The Action.SMSSend
class has a few more methods, which correspond to several useful functions of the Library. Let’s take a look at three of them.
How to set an SMS sender name?
SetSender("sender_name")
sets the SMS sender name, i.e., the text displayed on the SMS recipient’s mobile phone instead of the number. This can be the brand or a product name, something characteristic that people will associate with your company. You must agree that it looks better than an unknown phone number. It also helps draw attention, especially since we receive many mobile notifications daily.
You can create SMS sender names in the Customer Portal. From the programming perspective, these are handled by SenderFactory
and the actions from the Api/Action/Sender folder.
Managing SMS sender names in the SMSAPI C# library
Let’s create SMS sender names in the Customer Portal and manage them using the code. Do it using SenderFactory
and four actions: List, Add, SetDefault, and Delete. The simplest way is to handle them using the method from this factory.
The example below shows a listing of all sender names created, adding a new one, setting it up as the default, and deleting it.
var senderFactory = new SenderFactory(client);
var senderNamesResp = senderFactory.ActionList().Execute();
foreach (var sender in senderNamesResp.List){
System.Console.WriteLine(
"Name:"
+sender.Name
+", status: "
+sender.Status
+", dafault: "
+sender.Default);
}
senderFactory.ActionAdd("NewSenderName").Execute();
senderFactory.ActionSetDefault("NewSenderName").Execute();
senderFactory.ActionDelete("NewSenderName").Execute();
In the following article on SMS branding (link below), you’ll find more tips and requirements concerning SMS sender names. I’ll just mention here that for security reasons, our employee must approve each request. There’s more to it, so read on!
Scheduling SMS sending
An important matter when planning an SMS campaign is to put together a delivery schedule. When a notification reaches the client is essential for marketing effectiveness. SMS API enables you to set up the date and time of delivery.
The Action.SMSSend
class does this through the SetDateSent(DateTime data)
method. An argument is a standard System.DateTime
object. The following code will send an SMS on 14 November 2022 at 16:20:00 hours.
DateTime sendDate = new DateTime(2021, 9, 30,
16, 30, 0,
DateTimeKind.Local);
var sendResult = SMSFactory.ActionSend()
.SetTo("48111222333")
.SetText("Hello in the future!")
.SetDateSent(sendDate)
.Execute();
You can cancel a scheduled messaging using the SMSDelete
action if you need to change your plans. You need a unique SMS ID from the system included in reply to its sending. Continuing with the previous code, it could look like this:
SMSFactory.ActionDelete(sendResult.List[0].ID);
More on scheduling SMS sending
Customising SMS contents
To make a good impression on the recipient, treat them like individuals. For example, you may address them by their first name. And no, it doesn’t mean you must manually type in every notification.
Instead, you can customise your message content using dynamic parameters. The API enables using up to 4 parameters tagged within the text using special strings: [%1%], [%2%], [%3%] and [%4%]. Individual messages will be replaced with texts fed into the SetParam(int i, string text)
method. This is shown in the example:
SMSFactory.ActionSend()
.SetTo("48111222333", "48444555666")
.SetText("Hi [%1%], your no. is [%2%]!")
.SetParam(0,["Adam", "Bert"])
.SetParam(1,["11", "22"])
.Execute();
The two messages will look as follows:
"Hi Adam, your no. is 11!"
"Hi Bert, your no. is 22!"
With similarly constructed strings, you can use information from the contact database to send SMS using C#. This allows you to customise messages without putting parameter values in the code. For example:
SMSFactory.ActionSend()
.SetTo("48111222333", "48444555666")
.SetText("Hello [%name%], your number is [%1%]!")
.SetParam(0,["11", "22"])
.Execute();
The [%name%] field will be replaced with the relevant value assigned to the contact. You can find a list of standard fields in the bulk SMS sending documentation.
Handling SMS sending errors – when not everything runs smoothly
You can easily guess that errors in the C# library are handled by throwing exceptions. The Library defines several types. They contain a message and an error code. Find the error code list with descriptions in their Documentation section. Expect the following exceptions in the Visual Studio console:
ActionException
– different errors for individual actions. For example, code 11 means a message is too long or has no message content; 13 is an incorrect telephone number; 14 means an incorrect sender field; 26 is the message subject is too long.ClientExceptin
– HTTP client errors. The codes are, for example, 101 (incorrect token), 103 (insufficient points on account), and 105 (incorrect IP address).HostException
– server-side errors. Sample codes are 8 (call error) and 201 (internal system error).ProxyException
– these do not concern SMSAPI, but server connection, so they contain no codes. This exception is thrown in reaction to a Net.WebException.ArgumentException
– it’s an exception from the System space of the C# language. It’s thrown if you try to send an empty message or a recipient is a group and an individual number simultaneously (To and Group parameters rule each other out).
Other abilities of the C# SMS API library
As your business grows, you’ll probably expand the list of your projects to SMS notifications and marketing campaigns. If you happen to run into any problems, save yourself the trouble and chat with our support! You’ll find the link in the lower right corner of your screen.
Manage them manually in the Customer Portal, or better, in the code using ContactsFactory
. It provides many actions for listing, creating, editing and deleting contacts (and their individual fields) and whole groups. Take a look at the Action/Contacts and smsapiTests/Contacts folders in Visual Studio to learn about them in detail.
In addition to SMS, you can also send MMS messages. The Library supports them with MMSFactory
in a manner very similar to how you handle SMS messages. As with SMS, you can set up the recipient(s) and sending time. The response is of the same Response.Status
type as well. The content, of course, is not simple text but a message in the XML format SMIL, which you must first format correctly.
I hope you now know how to use C# application to run SMS campaigns. If you’re uncertain how to use the particular methods, study the examples in the test catalogue. You can also find explanations and program fragments in the API documentation. Good luck and successful texting using C#!