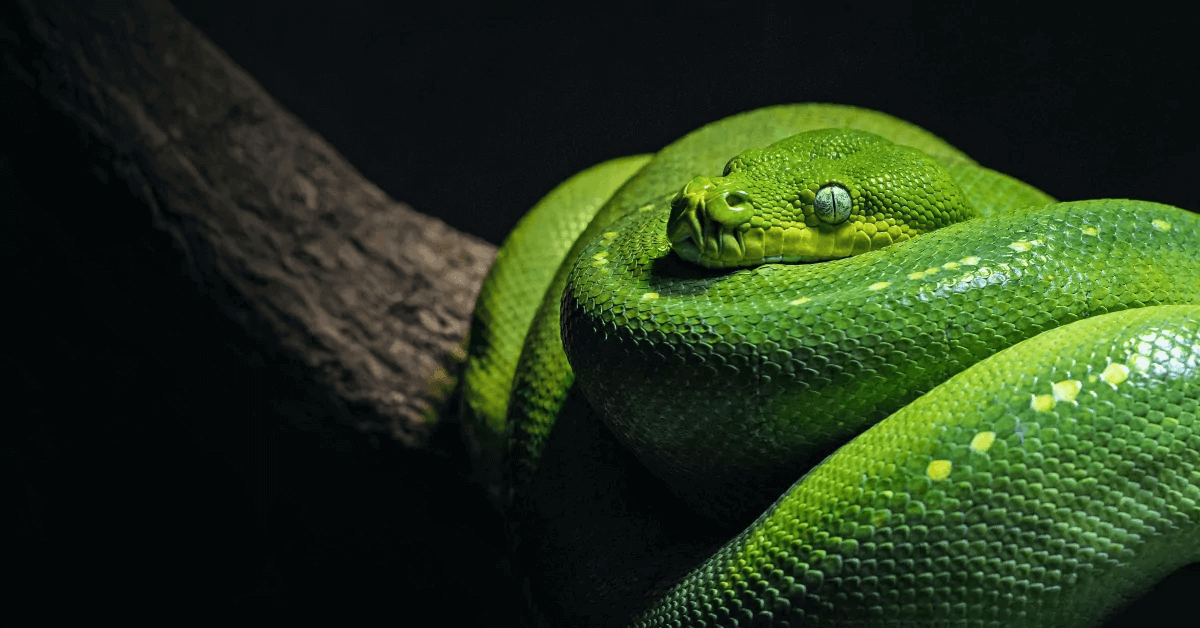
Table of Contents
And now for something completely different! The time has come to discuss another programming library for SMS campaigns. It is written in a peculiar but well-liked language. It’s something for those who enjoy British humour and fast scripts but detest braces. Meet our Python library!
First, let me direct you to the relevant Python SMS API repository on GitHub, where you can find the production code, tests and examples. Studying it file by file will not be necessary, though. Below I’ll discuss the essential features of the library, which will allow you to send SMS text messages using Python.
I will frequently reference the API Documentation, which describes communication between any application and the server. You are also welcome to read how to send SMS text messages from PHP, Java, JavaScript and C# over the internet.
The Python library: installing and configuring SMS sending
Before you begin coding, we need to prepare a few things. First of all, install the library. It is hosted outside GitHub on the PyPI website (Python Package Index). This makes for a lightning-fast installation using the pip package manager.
Installation instructions
Use the following command line to install the Python file in a few seconds:
pip install smsapi-client
As with the SMSAPI libraries in other languages, your program will communicate with the server using HTTP methods: GET, PUT, POST and DELETE. They will be handled by the external library Requests, which you must instal. It will work “behind the scenes” in the finished app, so no worries – you don’t need to be an expert in network protocols to manage SMS campaigns using software code.
Create SMSAPI account
There is one more thing you need to do to send SMS notifications in Python: create and configure your SMSAPI account! You’ll find a quick guide below:
This way, we will commence our cooperation, and you can use the entire SMS API. Also, remember to complete the necessary information:
- personal info: full name, e-mail address and password, phone number;
- your company data – for invoicing;
- SMS sender name, which you will read about later;
- also helpful: e-mail address for sending invoices and contact phone to your accounting department.
Next, in the Customer Panel, generate an OAuth 2 authentication token. It’s a sort of cryptographic API key. With it, the program can access the SMSAPI server resources. Paste the auth token into the script or place it in your configuration file.
Create a test account
We are SMSAPI – a global SMS messaging provider for companies, startups and the public sector. Create a free test account.
Watch the SMSAPI account tutorial:
Preparations are now complete! Feel free to sign in to the messaging gateway. Now let’s see what beginning to work with code in Python looks like.
The SMS library for Python applications
Python is liked for its simplicity. In this language, scripts can be clear and concise but at the same time advanced, and our library is no different. Simply ordering a single SMS to be sent looks like this:
from smsapi.client import SmsApiPlClient
token = '%SMSAPI_ACCESS_TOKEN%'
client = SmsApiPlClient(access_token=token)
result = client.sms.send(to='+44111222333', message='Hello world!')
But what exactly is going on here? First, a client instance is created, which manages the server connection – which is why you need the token. Formulating server queries belongs to API communication implementation, which the library takes care of for you.
The client will be used every time because it contains objects responsible for all available functions. The following line simply refers to the sms
object and orders the message to be sent to the given phone number. That’s it!
The send
function can accept multiple parameters, which modify the sending differently. They are described in the Documentation section on single SMS, and you can read about a few of the most useful ones later herein.
The returned value ‘result’ is an iterable object of the class SmsSendResult
, which contains the parameters of the messages sent. Specifically, these are count
, or the number of messages ordered for sending, and results
– a list of dictionaries that correspond to the individual ones. A dictionary contains, for example:
id
– a unique identifier of a message in our system;points
– cost, or the number of points deducted from your account;number
– recipient’s number;status
– delivery status (list of statuses in the Documentation)date_sent
– a time when the text was sent.
Optional parameters
The other fields of the SmsSendResult
are optional and are present if the details=1
parameter is used:
message
– the content of the text message;length
– message length;parts
– how many parts it is divided into.
Tutorial: how to send SMS messages using SMS API
Your bulk SMS campaigns wouldn’t be possible without the ability to send messages to multiple recipients simultaneously. Nothing could be simpler! Instead of a single phone number, you need to provide a list:
from smsapi.client import SmsApiPlClient
token = '%SMSAPI_ACCESS_TOKEN%'
client = SmsApiPlClient(access_token=token)
receivers = ['+44111222333', '+44444555666']
results = client.sms.send(to=receivers, message='Hello receivers!')
for result in results:
print(result)
As I’ve mentioned, the object returned by the sending order is iterable, so the above for loop will write the details of individual messages on the console.
In the Customer Portal, you’ll find the ability to create recipient groups, which streamlines organising and sending SMS in Python script. With another method, you can use this facility in the following code:
from smsapi.client import SmsApiPlClient
token = '%SMSAPI_ACCESS_TOKEN%'
client = SmsApiPlClient(access_token=token)
client.sms.send_to_group(
group='Group_name',
message='Hello group!')
The to
and group
parameters are directed to the same server query. However, they are mutually exclusive, and using them simultaneously will cause an API error. This is why the Python library has separate functions for them.
Additional features of the Python SMS gateway
As I’ve mentioned, messaging using Python can be modified by setting the delivery time, custom SMS sender name field, and custom content.
1. Schedule and send an SMS using Python
The right moment to deliver a text message is critical to drawing the recipient’s attention, more on which you can read in the article on planning SMS sending.
To make your campaign schedule happen, use the date
parameter. Scheduling messages to be sent on 1 December 2023 at 12:30 p.m. using a standard datetime
object looks like this:
from smsapi.client import SmsApiPlClient
from datetime import datetime
token = '%SMSAPI_ACCESS_TOKEN%'
client = SmsApiPlClient(access_token=token)
send_time = datetime(2023,12,1,12,30).isoformat()
response = client.sms.send(
to='+44111222333',
message='Hello in the future!',
date=send_time)
Accepted date formats are either Unix time (the number of seconds since 1 January 1970) or ISO 8601 (here: 2021-12-01T12:30:00+01:00).
The example below uses the latter option, thus the isoformat()
method used. If your plans change, you can cancel a scheduled message. To do this, you need its unique ID, which you should note in advance. Continuing from the previous listing:
smsIdToCancel = response.results[0].id
client.sms.remove_scheduled(smsIdToCancel)
2. SMS sender name instead of the phone number
Another vital feature is the SMS sender name. It is a short text that will be displayed instead of the actual phone numbers. It’s the name of a company, brand or product – something to let the recipient know who’s texting instantly.
This sort of “presentation” can effectively draw the prospective customer’s attention. Especially since many people receive several notifications on their phones every day. You can manage SMS senders in the relevant Sender names section in the Customer Panel. To use one of them in Python code, use the from parameter:
from smsapi.client import SmsApiPlClient
token = '%SMSAPI_ACCESS_TOKEN%'
client = SmsApiPlClient(access_token=token)
client.sms.send(
to='+44111222333',
message='Hello world!',
from='sender_name')
Handling SMS sender names using Python
As mentioned, SMS sender names displayed instead of phone numbers allow you to present yourself to the recipient. You can manage them manually in the Customer Portal or use software code in a script. This is made possible by the Sender
class, which provides the following methods:
add(name)
– adds a new sender name;check(name)
– returns an object with the status, name and flag that says if the name is the default;remove(name)
– deletes a name;default(name)
– sets a name as default;list()
– returns a list of all names.
This class is used in the same way as for sending text notifications. Here is what listing, adding a new name, setting it as default and verifying it looks like:
senderList = client.sender.list()
for senderName in senderList
print(senderName)
client.sender.add('NewSenderName')
client.sender.default('NewSenderName')
client.sender.check('NewSenderName')
Our employee verifies each new sender name to ensure that the name is not legally restricted. Add yours in the Customer Portal, sign the statement and wait for us to authorize it. You’ll get a notification when it’s ready to use.
Keep in mind that some countries limit the usage of this feature, so it’s best to contact your consultant to check all requirements. Other than that, there are technical limitations: maximum length and permitted characters, i.e., the GSM standard allows up to eleven alphanumeric characters. You can read more on that in the technical FAQ below.
3. Customising your SMS text messages
A third meaningful way to polish your SMS campaign, other than scheduling and sender IDs, is SMS customisation. It allows you to modify each text sent in bulk in a certain way. Individual recipients will then feel treated more personally.
In practice, it works using special character strings within a message. In individual SMS sent, these strings are replaced with the target text. This text, in turn, is drawn either from the contact database or from one of four custom parameters. See the latter case in the following simplified example:
receivers = ['+44111222333', '+44444555666']
client.sms.send(
to=receivers,
message='Hello [%1%], your number is [%2%]!',
param1=['Adam', 'Bert']
param2=['11', '22'])
In consecutive messages, field [%1%] will be replaced with successive elements of the param1 list, and similarly for [%2%] and the param2 list. In the end, the messages will look like this:
Hello Adam, your number is 11!
Hello Bert, your number is 22!
You can write it more elegantly using the contact database. Use the following commands:
receivers = ['+44111222333', '+44444555666']
client.sms.send(
to=receivers,
message='Hello [%imie%], your number is [%1%]!',
param1=['11', '22'])
In this case, the [%imie%] parameter will be replaced with the name associated with the specific recipient in the contact database. Of course, there are more standard fields: family name, e-mail, city, and others, all described in the Documentation.
4. SMS content templates for the Python app
SMS content templates provide make things somewhat easier when bulk messaging. They are message formulas that you can create in the templates tab. With them, you save time on writing identical notifications all over again.
If you also use parameters in them, what you get is modifiable content. You receive greater convenience at work and customised messages to boot. Using a sample template could look like this:
PackageSentTemplate: „Hi [%imie%], your order has been sent! It will arrive within [%1%] business days.”
receivers = ['+44111222333', '+44444555666']
client.sms.send(
to=receivers,
template='PackageSentTemplate',
param1='4')
Handling SMS API errors
Expecting the best is worth being ready for the worst, which is why exception handling is essential. When an error in processing a particular query occurs on the server side, information about this will be sent instead of the typical response. The library will process it and will raise one of the exceptions.
Five classes, all inheriting after Exception
, have been defined. They have the following hierarchy:
SmsApiException
– master class, allows handling all exceptions from the SMSAPI library. Contains the message and, optionally, a code.ClientException
– occurs if no authorisation data is provided.EndpointException
– exceptions reported from the server side – see the list below.SendException
– class assigned to the message send action. If incorrect phone numbers are used, contain a list of their details.ContactsException
– exceptions when handling the contact database using software code.
Common error codes
You will find a detailed list of codes and their explanations in the documentation. The most commons are:
- 11 – message too long or no message;
- 12 – the message is divided into more parts than the limit;
- 13 – incorrect recipient number;
- 14 – incorrect sender name;
- 26 – message subject is too long;
- 40 – no group of this name exists;
- 101 – incorrect authorisation token;
- 103 – insufficient funds on the account to send the message;
- 8, 201, 999 – internal server errors.
The EndpointException
exception occurs in two more situations. One is server communication errors. The code comes from the HTTP response, such as the familiar 404 error – no resource found.
The other possibility is using an unrecognised parameter name, most likely a typo. For example, write „massage” instead of „message”.
More options for handling mobile communication in Python
The capabilities of our library reach farther, though! Now that we’re near the end, it’s worth giving some of them the spotlight, such as SMS campaigns or contact database handling.
Sending MMS with Python
Sending MMS messages is important. Handling them is very simple:
response = client.mms.send(
to='+44111222333',
smil=SMIL_contents,
subject='Hello multimedia')
The mandatory smil parameter is a correct multimedia message in the XML format SMIL 1.0, which you must prepare beforehand. An object of the same type as SMS is returned: SmsSendResult
. Additionally, you can provide a text-based message subject
Managing contact databases
I’ve already mentioned the contact database in this article. When running marketing actions, having a list of prospective customers is crucial.
SMSAPI enables you to use a contact database to run an SMS campaign practically. You can manage such a database in the Customer Panel, but the Python library also allows you to do that.
The client
also has, in addition to the sms
, sender
and mms
objects, the contacts
instance. Therefore, the way you use the latter is similar to what I’ve shown you in the examples before. Creating a new contact, for example:
response = client.contacts.create_contact(
first_name='Dwayne',
last_name='Hicks',
phone_number='+44111222333',
email='dhicks@example.com',
gender='male'
birthday_date='2000-01-31')
The individual functions allow you to read, list, create, and delete individual contacts and whole groups. It is also possible to assign and remove a contact from a group.
The SMS API for Python: summary
And that’s it for the essential operation of SMS sending in Python! However, this article still hasn’t exhausted all the library capabilities. Feel free to test text and other features of SMSAPI: automated notification, receive messages, HLR lookup and more!
You can find more samples of how the SMS API gateway functions and features are used in the example folder on GitHub. The repository also includes a folder of unit tests written in the unittest framework. They can serve as a source of sample applications for different methods too.
How to handle incoming messages?
Outbound messaging (sending SMS) is nice and all, but what about inbound (receiving messages)? Nothing is easier than using SMS API to receive text messages using Python or any other language.
And the API Documentation, frequently referenced already, provides a source of knowledge on the meaning of individual parameters, statuses and codes. Now you have all the knowledge you need to integrate Python with SMS text messages. Off you go!